L06-异常处理
Java异常
在使用计算机语言进行项目开发的过程中,即使程序员把代码写得尽善尽美,在系统的运行过程中仍然会遇到一些问题,因为很多问题不是靠代码能够避免的,比如:客户输入数据的格式,读取文件是否存在,网络是否始终保持通畅等。
概述
异常:在Java语言中,将程序执行中发生的不正常情况称为“异常”。(开发过程中的语法错误和逻辑错误不是异常)
异常分类
Error: Java虚拟机无法解决的严重问题。如:JVM系统内部错误、资源耗尽等严重情况。一般不编写针对性的代码进行处理。
Exception: 其它因编程错误或偶然的外在因素导致的一般性问题,可以使用针对性的代码进行处理。例如:
- 空指针访问
- 试图读取不存在的文件
- 网络连接中断
解决方法
对于这些错误,一般有两种解决方法:
- 一是遇到错误就终止程序的运行。
- 另一种方法是由程序员在编写程序时,就考虑到错误的检测、错误消息的提示,以及错误的处理。
Java异常类层次
捕获错误最理想的是在编译期间,但有的错误只有在运行时才会发生。比如:除数为0,数组下标越界等。
分类:编译时异常和运行时异常
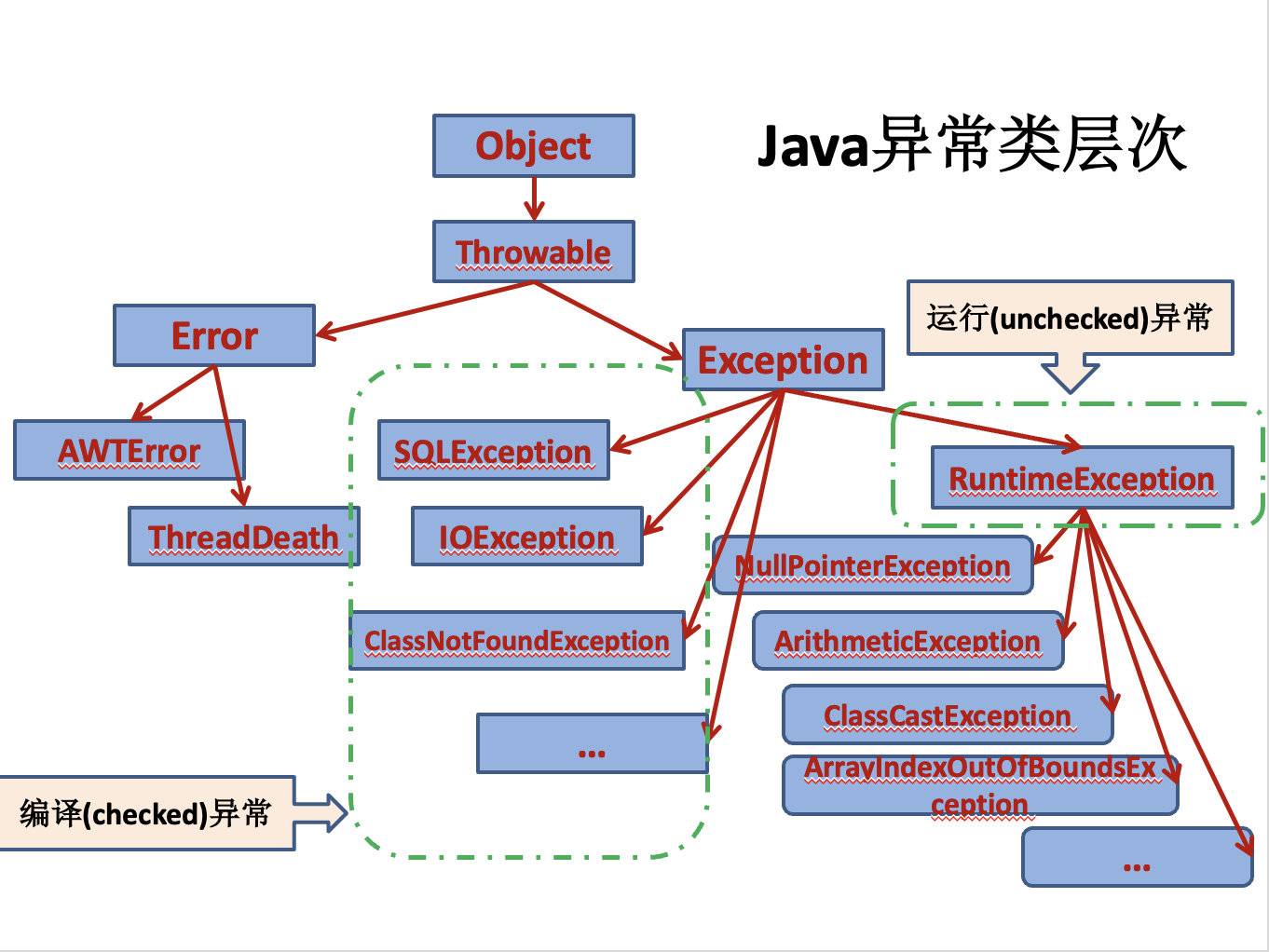
运行时异常
概述
是指编译器不要求强制处置的异常。一般是指编程时的逻辑错误,是程序员应该积极避免其出现的异常。java.lang.RuntimeException类及它的子类都是运行时异常。
对于这类异常,可以不作处理,因为这类异常很普遍,若全处理可能会对程序的可读性和运行效率产生影响。
ArrayIndexOutOfBoundsException
1 | // 数组下标越界异常:ArrayIndexOutOfBoundsException |
ArithmeticException
1 | // 算术异常:ArithmeticException |
ClassCastException
1 | // 类型转换异常:ClassCastException |
NullPointerException
1 | // 空指针异常:NullPointerException |
编译时异常
是指编译器要求必须处置的异常。即程序在运行时由于外界因素造成的一般性异常。编译器要求java程序必须捕获或声明所有编译时异常。
对于这类异常,如果程序不处理,可能会带来意想不到的结果。
异常处理机制
Java采用异常处理机制,将异常处理的程序代码集中在一起,与正常的程序代码分开,使得程序简洁,并易于维护。
抓抛模型
抛出(throw)异常
Java程序的执行过程中如出现异常,会生成一个异常类对象,该异常对象将被提交给Java运行时系统,这个过程称为抛出(throw)异常。
异常对象的生成:
- 由虚拟机自动生成:程序运行过程中,虚拟机检测到程序发生了问题,如果在当前代码中没有找到相应的处理程序,就会在后台自动创建一个对应异常类的实例对象并抛出——自动抛出
- 由开发人员手动创建:
Exception exception = new ClassCastException();
——创建好的异常对象不抛出对程序没有任何影响,和创建一个普通对象一样
捕获(catch)异常
如果一个方法内抛出异常,该异常对象会被抛给调用者方法中处理。如果异常没有在调用者方法中处理,它继续被抛给这个调用方法的上层方法。这个过程将一直继续下去,直到异常被处理。这一过程称为捕获(catch)异常。
- 如果一个异常回到main()方法,并且main()也不处理,则程序运行终止。
- 程序员通常只能处理Exception,而对Error无能为力。
try-catch-finally
异常处理是通过try-catch-finally语句实现的。
1 | try{ |
try
捕获异常的第一步是用try{…}
语句块选定捕获异常的范围,将可能出现异常的代码放在try语句块中。
catch(Exceptiontype e)
在catch语句块中是对异常对象进行处理的代码。每个try语句块可以伴随一个或多个catch语句,用于处理可能产生的不同类型的异常对象。
如果明确知道产生的是何种异常,可以用该异常类作为catch的参数;也可以用其父类作为catch的参数。比如:可以用ArithmeticException类作为参数的地方,就可以用RuntimeException类作为参数,或者用所有异常的父类Exception类作为参数。但不能是与ArithmeticException类无关的异常,如NullPointerException(catch中的语句将不会执行)。
捕获异常的有关信息:与其它对象一样,可以访问一个异常对象的成员变量或调用它的方法。
getMessage()
: 获取异常信息,返回字符串printStackTrace()
: 获取异常类名和异常信息,以及异常出现在程序中的位置,返回值void
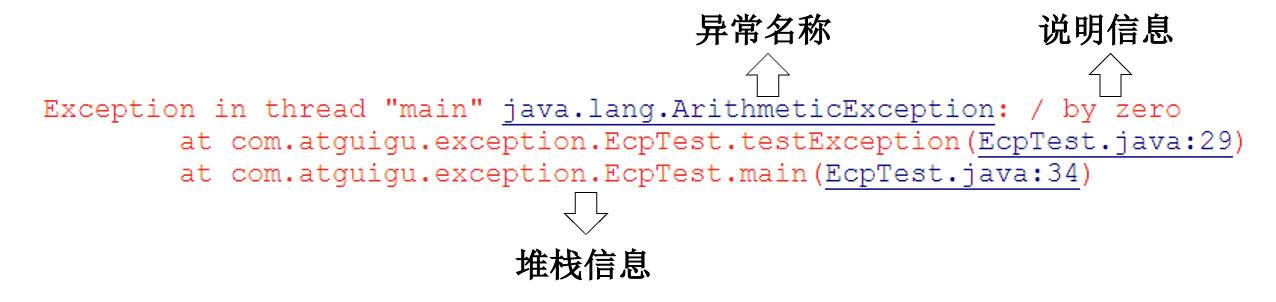
finally
- 捕获异常的最后一步是通过finally语句为异常处理提供一个统一的出口,使得在控制流转到程序的其它部分以前,能够对程序的状态作统一的管理。
- 不论在try代码块中是否发生了异常事件,catch语句是否执行,catch语句是否有异常,catch语句中是否有return,finally块中的语句都会被执行。
- finally语句和catch语句是任选的。
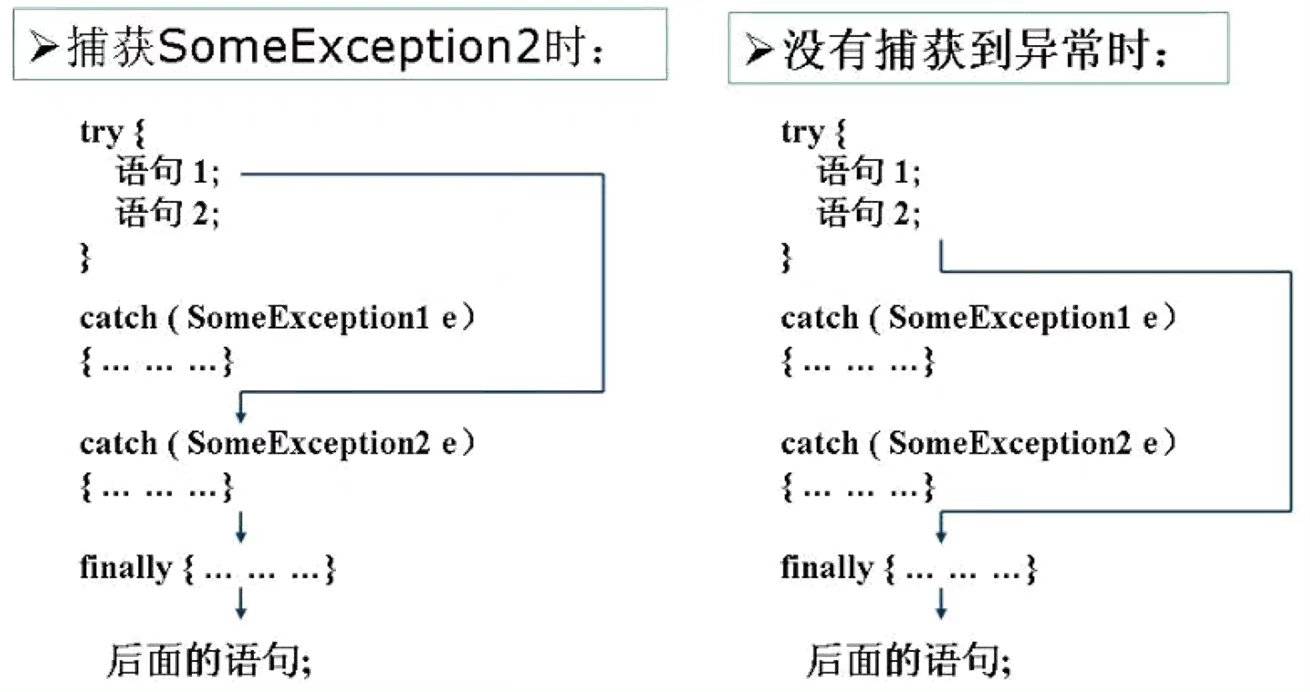
练习
练习:编写一个类TestException,在main方法中使用try、catch、finally,要求:
- 在try块中,编写被零除的代码
- 在catch块中,捕获被零除所产生的异常,并且打印异常信息
- 在finally块中,打印一条语句
1 | package L06.exer2; |
声明抛出异常
声明抛出异常是Java中处理异常的第二种方式:
如果一个方法(中的语句执行时)可能生成某种异常,但是并不能确定如何处理这种异常,则此方法应显式地声明抛出异常,表明该方法将不对这些异常进行处理,而由该方法的调用者负责处理。
在方法声明中用throws语句可以声明抛出异常的列表,throws后面的异常类型可以是方法中产生的异常类型,也可以是它的父类。
示例
1 | public void readFile(String file) throws FileNotFoundException { |
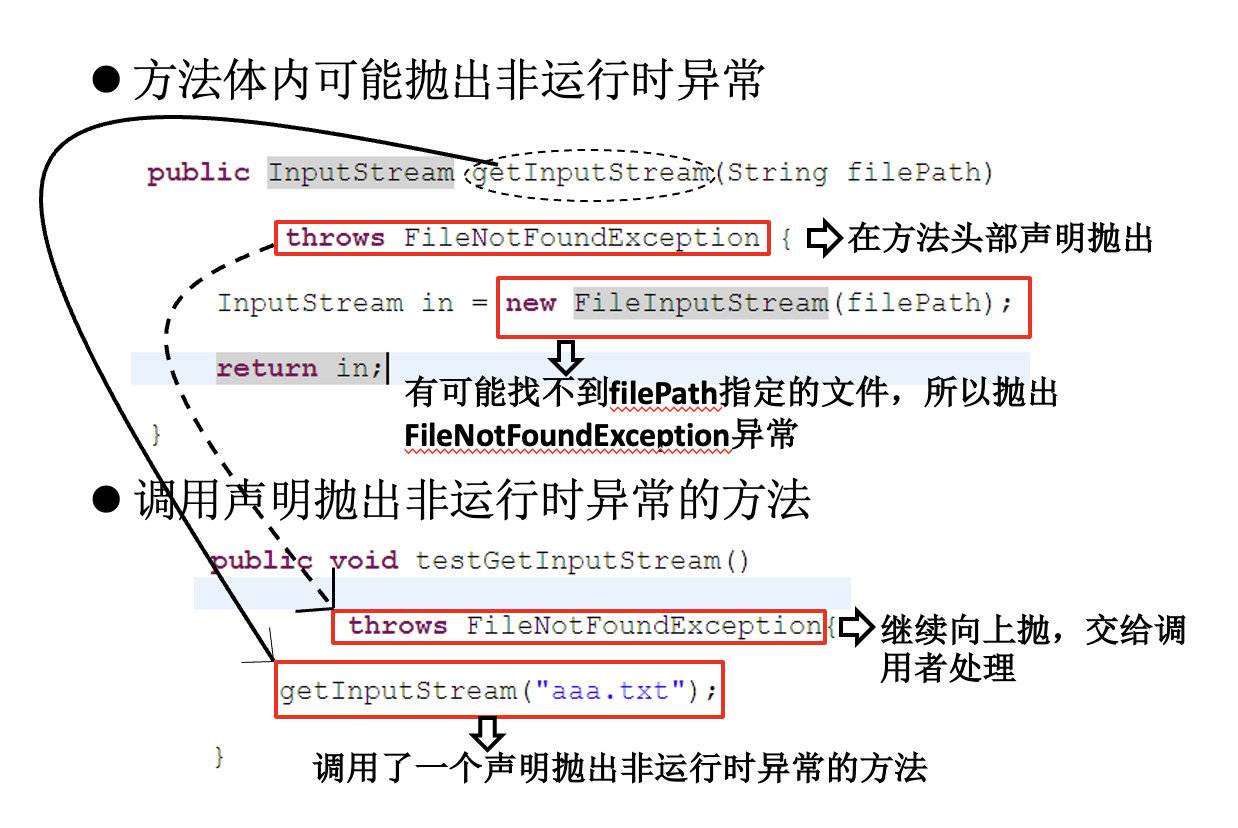
- 重写方法声明抛出异常的原则
重写方法不能抛出比被重写方法范围更大的异常类型。在多态的情况下,对methodA()方法的调用-异常的捕获按父类声明的异常处理。
1 | public class A { |
人工抛出异常
Java异常类对象除在程序执行过程中出现异常时由系统自动生成并抛出,也可根据需要人工创建并抛出。
首先要生成异常类对象,然后通过throw语句实现抛出操作(提交给Java运行环境)。
1
2IOException e = new IOException();
throw e;可以抛出的异常必须是Throwable或其子类的实例。下面的语句在编译时将会产生语法错误:
1
throw new String("want to throw");
自定义异常类
概述
- 一般地,用户自定义异常类都是RuntimeException的子类。
- 自定义异常类通常需要编写几个重载的构造器。
- 自定义的异常类对象通过throw抛出。
- 自定义异常最重要的是异常类的名字,当异常出现时,可以根据名字判断异常类型。
创建
用户自定义异常类MyException,用于描述数据取值范围错误信息。用户自己的异常类必须继承现有的异常类。
1 | class MyException extends Exception { |
使用
1 | public class Test{ |
总结
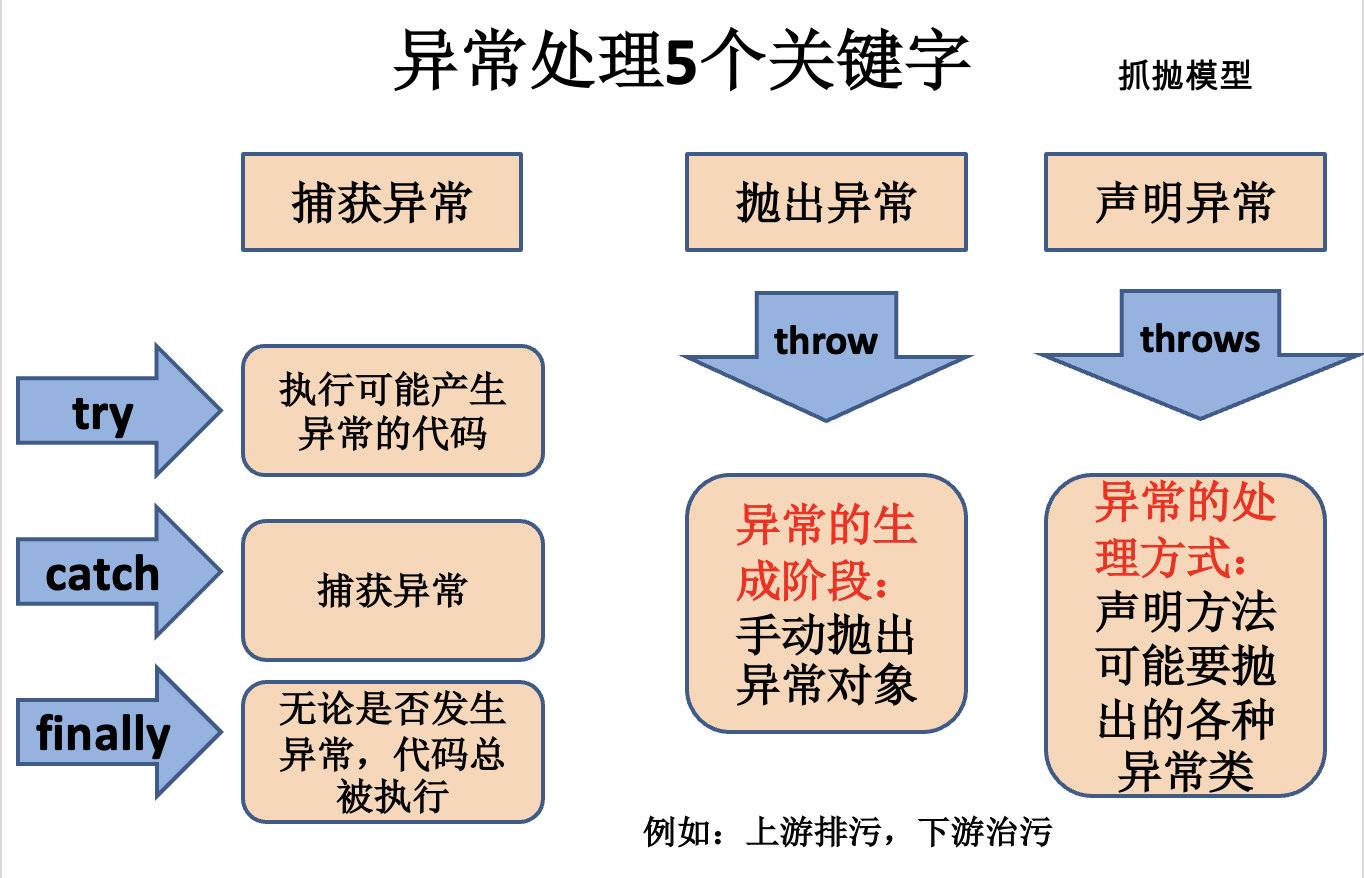
练习
编写应用程序EcmDef.java,接收命令行的两个参数,要求不能输入负数,计算两数相除。 对数据类型不一致(NumberFormatException)、缺少命令行参数(ArrayIndexOutOfBoundsException)、除0(ArithmeticException)及输入负数(EcDef 自定义的异常)进行异常处理。
- 在主类(EcmDef)中定义异常方法(ecm)完成两数相除功能。
- 在main()方法中使用异常处理语句进行异常处理。
- 在程序中,自定义对应输入负数的异常类(EcDef)。
- 运行时接受参数 java EcmDef 20 10 。
//args[0]=“20” args[1]=“10”
- Interger类的static方法parseInt(String s)将s转换成对应的int值。如
int a=Interger.parseInt(“314”); //a=314;
1 | package L06.exer3; |
面试题
- 运行时异常与一般异常有何异同
答:异常表示程序运行过程中可能出现的非正常状态,运行时异常表示虚拟机的通常操作中可能遇到的异常,是一种常见运行错误。java编译器要求方法必须声明抛出可能发生的非运行时异常,但是并不要求必须声明抛出未被捕获的运行时异常。
- Java中的异常处理机制的简单原理和应用
答:当JAVA程序违反了JAVA的语义规则时,JAVA虚拟机就会将发生的错误表示为一个异常。违反语义规则包括2种情况。一种是JAVA类库内置的语义检查。例如:数组下标越界,会引发IndexOutOfBoundsException;访问null的对象时会引发NullPointerException。另一种情况就是JAVA允许程序员扩展这种语义检查,程序员可以创建自己的异常,并自由选择在何时用throw关键字引发异常。所有的异常都是java.lang.Thowable的子类。
垃圾回收的优点和原理,并考虑2种回收机制
答:Java语言中一个显著的特点就是引入了垃圾回收机制,使c++程序员最头疼的内存管理的问题迎刃而解,它使得Java程序员在编写程序的时候不再需要考虑内存管理。由于有个垃圾回收机制,Java中的对象不再有”作用域”的概念,只有对象的引用才有”作用域”。垃圾回收可以有效的防止内存泄露,有效的使用可以使用的内存。垃圾回收器通常是作为一个单独的低级别的线程运行,不可预知的情况下对内存堆中已经死亡的或者长时间没有使用的对象进行清楚和回收,程序员不能实时的调用垃圾回收器对某个对象或所有对象进行垃圾回收。回收机制有分代复制垃圾回收和标记垃圾回收,增量垃圾回收。JAVA语言如何进行异常处理,关键字:throws、throw、try、catch、finally分别代表什么意义?在try块中可以抛出异常吗?
答:Java通过面向对象的方法进行异常处理,把各种不同的异常进行分类,并提供了良好的接口。在Java中,每个异常都是一个对象,它是Throwable类或其它子类的实例。当一个方法出现异常后便抛出一个异常对象,该对象中包含有异常信息,调用这个对象的方法可以捕获到这个异常并进行处理。Java的异常处理是通过5个关键词来实现的:try、catch、throw、throws和finally。一般情况下是用try来执行一段程序,如果出现异常,系统会抛出(throws)一个异常,这时候你可以通过它的类型来捕捉(catch)它,或最后(finally)由缺省处理器来处理。
用try来指定一块预防所有”异常”的程序。紧跟在try程序后面,应包含一个catch子句来指定你想要捕捉的”异常”的类型。throw语句用来明确地抛出一个”异常”。throws用来标明一个成员函数可能抛出的各种”异常”。Finally为确保一段代码不管发生什么”异常”都被执行一段代码。
可以在一个成员函数调用的外面写一个try语句,在这个成员函数内部写另一个try语句保护其他代码。每当遇到一个try语句,”异常”的框架就放到堆栈上面,直到所有的try语句都完成。如果下一级的try语句没有对某种”异常”进行处理,堆栈就会展开,直到遇到有处理这种”异常”的try语句。
写出程序结果
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20class Demo{
public static void func(){
try{
throw new Exception();
}
finally{
System.out.println("B");
}
}
public static void main(String[] args){
try{
func();
System.out.println("A");
}
catch(Exception e){
System.out.println("C");
}
System.out.println("D");
}
} //编译不通过
编译失败
如果func放上声明了该异常。结果是?B C D
写出程序结果
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18class Demo{
public static void main(String[] args){
try{
showExce();
System.out.println("A");
}
catch(Exception e){
System.out.println("B");
}
finally{
System.out.println("C");
}
System.out.println("D");
}
public static void showExce() throws Exception{
throw new Exception();
}
} // BCD
写出程序结果
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20class Demo{
public static void func(){
try{
throw new Exception();
System.out.println("A");
}
catch(Exception e){
System.out.println("B");
}
}
public static void main(String[] args){
try{
func();
}
catch(Exception e){
System.out.println("C");
}
System.out.println("D");
}
}
// 编译失败。 因为打印“A”的输出语句执行不到。throw单独存在,下面不要定义语句,因为执行不到。
写出程序结果
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16class Exc0 extends Exception{}
class Exc1 extends Exc0{}
class Demo{
public static void main(String[] args){
try{
throw new Exc1();
}
catch(Exception e){
System.out.println("Exception");
}
catch(Exc0 e){
System.out.println("Exc0");
}
}
}
编译不通过!多个catch时,父类的catch要放在下面。
写出程序结果
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24class Test{
public static String output="";
public static void foo(int i){
try{
if(i==1)
throw new Exception();
output+="1";
}
catch(Exception e){
output+="2";
//return;
}
finally{
output+="3";
}
output+="4";
}
public static void main(String args[]){
foo(0);
System.out.println(output);//134
foo(1);
System.out.println(output);// 234
}
}
1 | public class ReturnExceptionDemo { |
- try { }里有一个return语句,那么紧跟在这个try后的finally {}里的code会不会被执行,什么时候被执行,在return前还是后?
答:会执行,在return前执行
- 给我一个你最常见到的RuntimeException
答:常见的运行时异常有如下这些ArithmeticException, ArrayStoreException, BufferOverflowException, BufferUnderflowException, CannotRedoException, CannotUndoException, ClassCastException, CMMException, ConcurrentModificationException,DOMException, EmptyStackException, IllegalArgumentException, IllegalMonitorStateException, IllegalPathStateException, IllegalStateException, ImagingOpException, IndexOutOfBoundsException, MissingResourceException, NegativeArraySizeException, NoSuchElementException, NullPointerException, ProfileDataException, ProviderException, RasterFormatException, SecurityException, SystemException, UndeclaredThrowableException, UnmodifiableSetException, UnsupportedOperationException
- Error和Exception有什么区别
答:Error 表示恢复不是不可能但很困难的情况下的一种严重问题。比如说内存溢出。不可能指望程序能处理这样的情况;Exception 表示一种设计或实现问题。也就是说,它表示如果程序运行正常,从不会发生的情况。
1 | public int aaa(){ |
打印结果是?——2
考虑把最后的return x写到finally里面结果就不同了。是3。